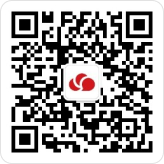
Python自动化代码库:Excel/爬虫/邮件告警场景模板即用
2025/03/17
从文件处理到Web操作,Python凭借丰富的库生态成为自动化任务的首选工具。以下是 办公、数据、运维、测试 四大领域的自动化解决方案,附可直接复用的代码模板。
一、文件与办公自动化
场景1:批量重命名文件
import os def batch_rename(path, prefix): for count, filename in enumerate(os.listdir(path)): new_name = f"{prefix}_{count+1}{os.path.splitext(filename)[1]}" os.rename( os.path.join(path, filename), os.path.join(path, new_name) print(f"Renamed: {filename} → {new_name}") batch_rename("/docs/photos", "vacation")
库推荐:os(系统操作)、shutil(高级文件操作)
场景2:Excel数据合并与清洗
import pandas as pd # 合并多个Excel文件 files = ["sales_2023Q1.xlsx", "sales_2023Q2.xlsx"] df = pd.concat([pd.read_excel(f) for f in files]) # 删除空值并保存 df.dropna(inplace=True) df.to_excel("merged_sales.xlsx", index=False)
库推荐:pandas(数据处理)、openpyxl(Excel操作)
二、网络与Web自动化
场景3:网页数据抓取
import requests from bs4 import BeautifulSoup url = "https://news.ycombinator.com" response = requests.get(url) soup = BeautifulSoup(response.text, "html.parser") # 提取标题和链接 for item in soup.select(".titleline > a"): print(item.text, item["href"])
库推荐:requests(HTTP请求)、BeautifulSoup4(HTML解析)
场景4:自动填写表单(Selenium)
from selenium import webdriver from selenium.webdriver.common.by import By driver = webdriver.Chrome() driver.get("https://example.com/login") # 输入用户名密码并提交 driver.find_element(By.ID, "username").send_keys("admin") driver.find_element(By.ID, "password").send_keys("pass123") driver.find_element(By.TAG_NAME, "button").click() driver.quit()
库推荐:selenium(浏览器自动化)、webdriver-manager(驱动管理)
三、系统与运维自动化
场景5:服务器监控告警
import psutil import smtplib # 获取CPU和内存使用率 cpu_usage = psutil.cpu_percent(interval=1) mem_usage = psutil.virtual_memory().percent if cpu_usage > 90 or mem_usage > 90: # 发送邮件告警 server = smtplib.SMTP('smtp.gmail.com', 587) server.starttls() server.login("your_email@gmail.com", "password") server.sendmail("from@example.com", "admin@example.com", f"Subject: Server Alert\n\nCPU: {cpu_usage}%, Memory: {mem_usage}%")
库推荐:psutil(系统监控)、smtplib(邮件发送)
场景6:批量部署SSH命令
import paramiko def run_ssh_command(host, username, password, command): client = paramiko.SSHClient() client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) client.connect(host, username=username, password=password) stdin, stdout, stderr = client.exec_command(command) print(stdout.read().decode()) client.close() # 在多台服务器执行更新 servers = ["192.168.1.101", "192.168.1.102"] for server in servers: run_ssh_command(server, "root", "password123", "apt update && apt upgrade -y")
库推荐:paramiko(SSH连接)
四、测试与质量保障
场景7:自动化单元测试
import unittest def add(a, b): return a + b class TestMath(unittest.TestCase): def test_add(self): self.assertEqual(add(2, 3), 5) self.assertRaises(TypeError, add, "2", 3) if __name__ == "__main__": unittest.main()
库推荐:unittest(测试框架)、pytest(更简洁的测试)
场景8:API接口自动化测试
import requests import json def test_api(): url = "https://api.example.com/login" payload = {"username": "test", "password": "123"} response = requests.post(url, json=payload) assert response.status_code == 200 assert json.loads(response.text)["token"] is not None test_api()
库推荐:requests(API调用)、pytest(断言优化)
五、进阶自动化工具
场景9:任务调度(定时执行)
from apscheduler.schedulers.blocking import BlockingScheduler def job(): print("定时任务执行中...") scheduler = BlockingScheduler() scheduler.add_job(job, 'cron', hour=8) # 每天8点执行 scheduler.start()
库推荐:APScheduler(任务调度)
场景10:GUI自动化(桌面应用控制)
import pyautogui # 打开记事本并输入文字 pyautogui.hotkey('win', 'r') pyautogui.write('notepad') pyautogui.press('enter') pyautogui.sleep(1) pyautogui.write('Hello, Python Automation!')
库推荐:pyautogui(GUI控制)、PyGetWindow(窗口管理)
自动化最佳实践
异常处理:使用try-except捕获并记录错误:
try: # 可能出错的代码 except Exception as e: print(f"Error: {e}") # 发送告警或回滚操作
日志记录:通过logging模块追踪任务状态:
import logging logging.basicConfig(filename='automation.log', level=logging.INFO) logging.info("任务开始执行")
性能优化:
多线程:使用threading并行处理任务
异步IO:asyncio提升网络请求效率
工具链推荐
用途 | 工具/库 | 优势 |
---|---|---|
数据可视化 | matplotlib/plotly | 生成动态图表 |
文档自动化 | python-docx/reportlab | 创建Word/PDF文件 |
数据库自动化 | sqlalchemy | ORM支持多数据库 |
命令行工具开发 | click | 快速构建CLI应用 |
Python官方文档的网址是:Python官方文档
-
开设课程 开班时间 在线报名OCP2025.04.26
在线报名
HCIP-AI Solution2025.04.26在线报名
HCIE-openEuler2025.05.03在线报名
RHCA-CL2602025.05.04在线报名
HCIP-Cloud2025.05.10在线报名
PGCM直通车2025.05.10在线报名
HCIA-Datacom(晚班)2025.05.19在线报名
HCIA-Sec2025.06.07在线报名
RHCA-RH4422025.06.07在线报名
PMP2025.06.10在线报名
HCIA-Datacom2025.06.14在线报名
HCIE-AI Solution2025.06.14在线报名
HCIE-Datacom2025.06.14在线报名
HCIP-Datacom(晚班)2025.06.16在线报名
OCM2025.06.21在线报名
HCIE-Cloud2025.06.21在线报名
HCIP-Sec2025.06.21在线报名
HCIE-Bigdata2025.06.28在线报名
RHCE2025.06.28在线报名
HCIE-Datacom考前辅导2025.07.05在线报名
HCIP-Datacom深圳2025.07.19在线报名
CISP2025.07.19在线报名
HCIA-Datacom(晚班)2025.07.21在线报名
RHCA-RH4362025.07.26在线报名
OCP2025.07.26在线报名
HCIE-Sec2025.08.09在线报名
HCIA-AI Solution2025.08.16在线报名
HCIP-Datacom(晚班)2025.08.25在线报名
RHCA-RH3582025.09.06在线报名
PMP2025.09.16在线报名
HCIE-Datacom2025.09.06在线报名
HCIA-AI Solution2025.09.27在线报名
HCIA-Datacom2025.09.27在线报名
PGCM直通车2025.10.11在线报名
RHCA-DO3742025.10.11在线报名
HCIA-Sec2025.10.11在线报名
RHCE2025.10.18在线报名
HCIP-Datacom2025.11.08在线报名
HCIP-Sec2025.11.08在线报名
RHCA-CL2602025.11.15在线报名
OCP2025.11.15在线报名
HCIE-Sec2025.12.13在线报名
HCIE-Datacom2026.01.10在线报名
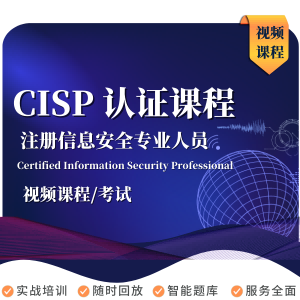
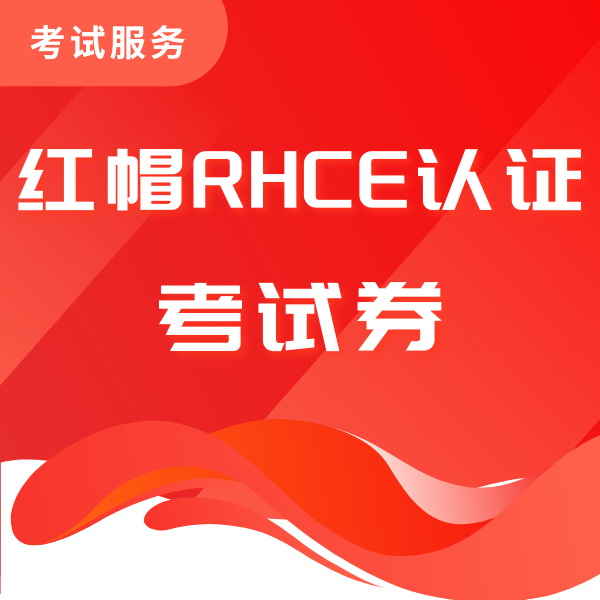
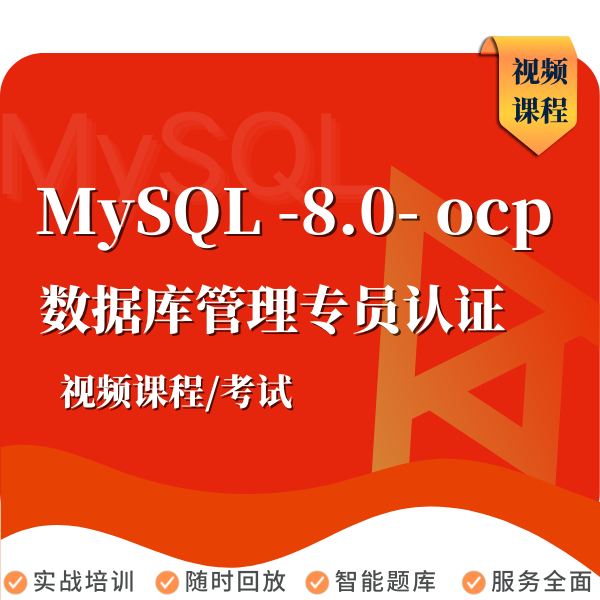
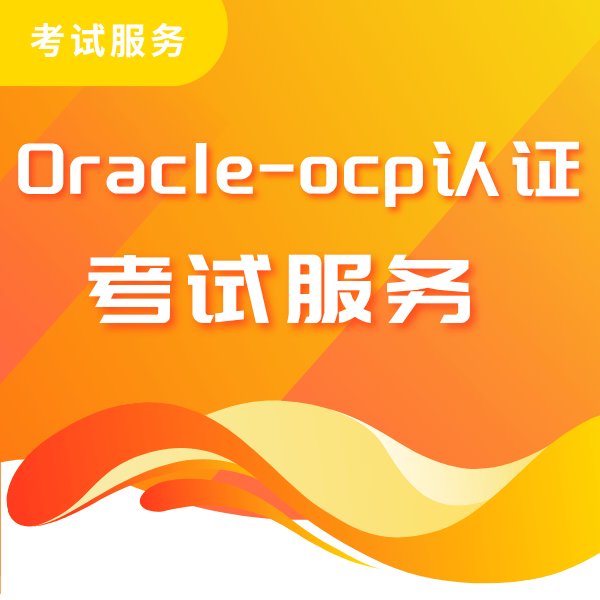